1. 用函數(shù)創(chuàng)建多線程
在Python3中,Python提供了一個(gè)內(nèi)置模塊 threading.Thread
,可以很方便地讓我們創(chuàng)建多線程。
threading.Thread()
一般接收兩個(gè)參數(shù):
線程函數(shù)名:要放置線程讓其后臺(tái)執(zhí)行的函數(shù),由我們自已定義,注意不要加()
;
線程函數(shù)的參數(shù):線程函數(shù)名所需的參數(shù),以元組的形式傳入。若不需要參數(shù),可以不指定。
舉個(gè)例子
import time
from threading import Thread
# 自定義線程函數(shù)。
def target(name="Python"):
for i in range(2):
print("hello", name)
time.sleep(1)
# 創(chuàng)建線程01,不指定參數(shù)
thread_01 = Thread(target=target)
# 啟動(dòng)線程01
thread_01.start()
# 創(chuàng)建線程02,指定參數(shù),注意逗號(hào)
thread_02 = Thread(target=target, args=("MING",))
# 啟動(dòng)線程02
thread_02.start()
可以看到輸出
hello Python
hello MING
hello Python
hello MING
2. 用類(lèi)創(chuàng)建多線程
相比較函數(shù)而言,使用類(lèi)創(chuàng)建線程,會(huì)比較麻煩一點(diǎn)。
首先,我們要自定義一個(gè)類(lèi),對(duì)于這個(gè)類(lèi)有兩點(diǎn)要求,
必須繼承 threading.Thread
這個(gè)父類(lèi);
必須復(fù)寫(xiě) run
方法。
這里的 run
方法,和我們上面線程函數(shù)
的性質(zhì)是一樣的,可以寫(xiě)我們的業(yè)務(wù)邏輯程序。在 start()
后將會(huì)調(diào)用。
來(lái)看一下例子 為了方便對(duì)比,run
函數(shù)我復(fù)用上面的main
。
import time
from threading import Thread
class MyThread(Thread):
def __init__(self, type="Python"):
# 注意:super().__init__() 必須寫(xiě)
# 且最好寫(xiě)在第一行
super().__init__()
self.type=type
def run(self):
for i in range(2):
print("hello", self.type)
time.sleep(1)
if __name__ == '__main__':
# 創(chuàng)建線程01,不指定參數(shù)
thread_01 = MyThread()
# 創(chuàng)建線程02,指定參數(shù)
thread_02 = MyThread("MING")
thread_01.start()
thread_02.start()
當(dāng)然結(jié)果也是一樣的。
hello Python
hello MING
hello Python
hello MING
3. 線程對(duì)象的方法
上面介紹了當(dāng)前 Python 中創(chuàng)建線程兩種主要方法。
創(chuàng)建線程是件很容易的事,但要想用好線程,還需要學(xué)習(xí)線程對(duì)象的幾個(gè)函數(shù)。
經(jīng)過(guò)我的總結(jié),大約常用的方法有如下這些:
# 如上所述,創(chuàng)建一個(gè)線程
t=Thread(target=func)
# 啟動(dòng)子線程
t.start()
# 阻塞子線程,待子線程結(jié)束后,再往下執(zhí)行
t.join()
# 判斷線程是否在執(zhí)行狀態(tài),在執(zhí)行返回True,否則返回False
t.is_alive()
t.isAlive()
# 設(shè)置線程是否隨主線程退出而退出,默認(rèn)為False
t.daemon = True
t.daemon = False
# 設(shè)置線程名
t.name = "My-Thread"
審核編輯:符乾江
-
多線程
+關(guān)注
關(guān)注
0文章
278瀏覽量
19956 -
python
+關(guān)注
關(guān)注
56文章
4797瀏覽量
84688
發(fā)布評(píng)論請(qǐng)先 登錄
相關(guān)推薦
socket 多線程編程實(shí)現(xiàn)方法
Python中多線程和多進(jìn)程的區(qū)別
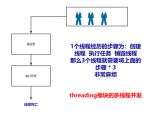
從多線程設(shè)計(jì)模式到對(duì) CompletableFuture 的應(yīng)用
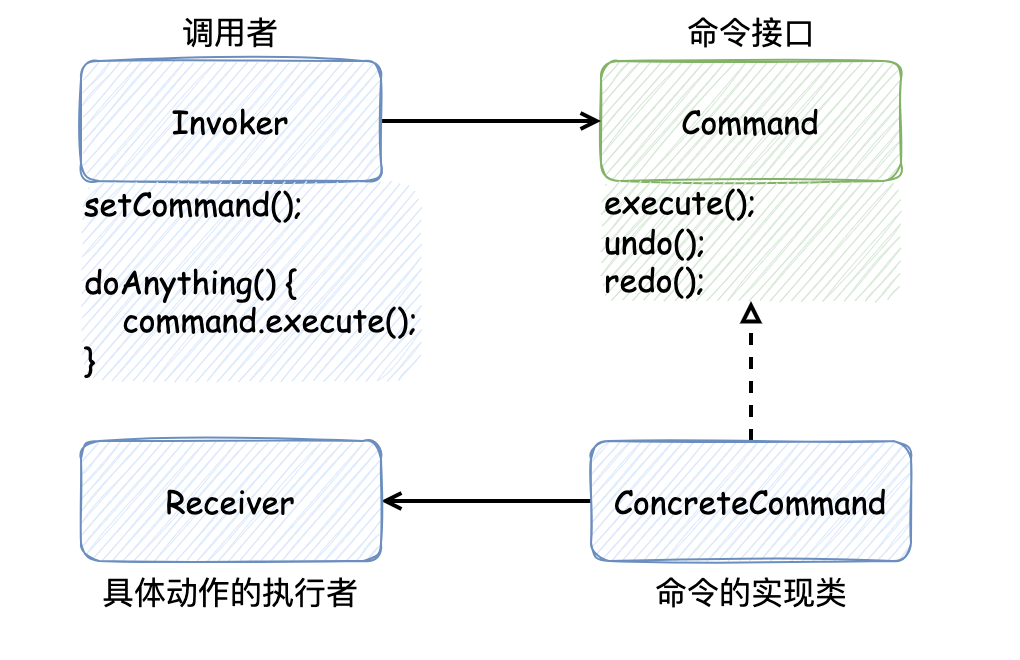
數(shù)字設(shè)備中采集數(shù)字圖像的兩種方法是什么
bootloader開(kāi)多線程做引導(dǎo)程序,跳app初始化后直接進(jìn)hardfualt,為什么?
鴻蒙原生應(yīng)用開(kāi)發(fā)-ArkTS語(yǔ)言基礎(chǔ)類(lèi)庫(kù)多線程并發(fā)概述
鴻蒙APP開(kāi)發(fā):【ArkTS類(lèi)庫(kù)多線程】TaskPool和Worker的對(duì)比(2)
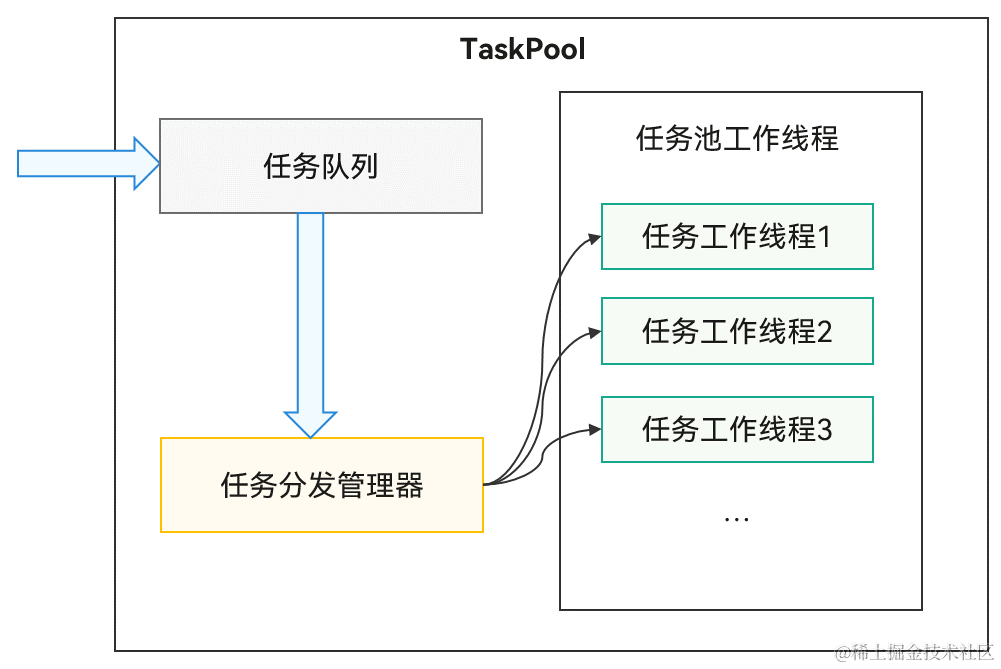
鴻蒙原生應(yīng)用開(kāi)發(fā)-ArkTS語(yǔ)言基礎(chǔ)類(lèi)庫(kù)多線程TaskPool和Worker的對(duì)比(一)
鴻蒙原生應(yīng)用開(kāi)發(fā)-ArkTS語(yǔ)言基礎(chǔ)類(lèi)庫(kù)多線程I/O密集型任務(wù)開(kāi)發(fā)
java實(shí)現(xiàn)多線程的幾種方式
python中5種線程鎖盤(pán)點(diǎn)
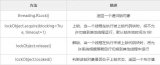
評(píng)論