1. context 介紹
很多時(shí)候,我們會(huì)遇到這樣的情況,上層與下層的goroutine需要同時(shí)取消,這樣就涉及到了goroutine間的通信。在Go中,推薦我們以通信的方式共享內(nèi)存,而不是以共享內(nèi)存的方式通信。
所以,就需要用到channl,但是,在上述場(chǎng)景中,如果需要自己去處理channl的業(yè)務(wù)邏輯,就會(huì)有很多費(fèi)時(shí)費(fèi)力的重復(fù)工作,因此,context出現(xiàn)了。
context是Go中用來(lái)進(jìn)程通信的一種方式,其底層是借助channl與snyc.Mutex實(shí)現(xiàn)的。
2. 基本介紹
context的底層設(shè)計(jì),我們可以概括為1個(gè)接口,4種實(shí)現(xiàn)與6個(gè)方法。
1 個(gè)接口
Context 規(guī)定了context的四個(gè)基本方法
4 種實(shí)現(xiàn)
emptyCtx 實(shí)現(xiàn)了一個(gè)空的context,可以用作根節(jié)點(diǎn)
cancelCtx 實(shí)現(xiàn)一個(gè)帶cancel功能的context,可以主動(dòng)取消
timerCtx 實(shí)現(xiàn)一個(gè)通過(guò)定時(shí)器timer和截止時(shí)間deadline定時(shí)取消的context
valueCtx 實(shí)現(xiàn)一個(gè)可以通過(guò) key、val 兩個(gè)字段來(lái)存數(shù)據(jù)的context
6 個(gè)方法
Background 返回一個(gè)emptyCtx作為根節(jié)點(diǎn)
TODO 返回一個(gè)emptyCtx作為未知節(jié)點(diǎn)
WithCancel 返回一個(gè)cancelCtx
WithDeadline 返回一個(gè)timerCtx
WithTimeout 返回一個(gè)timerCtx
WithValue 返回一個(gè)valueCtx
3. 源碼分析
3.1 Context 接口
type Context interface { Deadline() (deadline time.Time, ok bool) Done() <-chan struct{} Err() error Value(key interface{}) interface{} }
Deadline() :返回一個(gè)time.Time,表示當(dāng)前Context應(yīng)該結(jié)束的時(shí)間,ok則表示有結(jié)束時(shí)間
Done():返回一個(gè)只讀chan,如果可以從該 chan 中讀取到數(shù)據(jù),則說(shuō)明 ctx 被取消了
Err():返回 Context 被取消的原因
Value(key):返回key對(duì)應(yīng)的value,是協(xié)程安全的
3.2 emptyCtx
type emptyCtx int func (*emptyCtx) Deadline() (deadline time.Time, ok bool) { return } func (*emptyCtx) Done() <-chan struct{} { return nil } func (*emptyCtx) Err() error { return nil } func (*emptyCtx) Value(key interface{}) interface{} { return nil }
emptyCtx實(shí)現(xiàn)了空的Context接口,其主要作用是為Background和TODO這兩個(gè)方法都會(huì)返回預(yù)先初始化好的私有變量background和todo,它們會(huì)在同一個(gè) Go 程序中被復(fù)用:
var ( background = new(emptyCtx) todo = new(emptyCtx) ) func Background() Context { return background } func TODO() Context { return todo }
Background和TODO在實(shí)現(xiàn)上沒(méi)有區(qū)別,只是在使用語(yǔ)義上有所差異:
Background是上下文的根節(jié)點(diǎn);
TODO應(yīng)該僅在不確定應(yīng)該使用哪種上下文時(shí)使用;
3.3 cancelCtx
cancelCtx實(shí)現(xiàn)了canceler接口與Context接口: type canceler interface { cancel(removeFromParent bool, err error) Done() <-chan struct{} }
其結(jié)構(gòu)體如下:
type cancelCtx struct { // 直接嵌入了一個(gè) Context,那么可以把 cancelCtx 看做是一個(gè) Context Context mu sync.Mutex // protects following fields done atomic.Value // of chan struct{}, created lazily, closed by first cancel call children map[canceler]struct{} // set to nil by the first cancel call err error // set to non-nil by the first cancel call }
我們可以使用WithCancel的方法來(lái)創(chuàng)建一個(gè)cancelCtx:
func WithCancel(parent Context) (ctx Context, cancel CancelFunc) { if parent == nil { panic("cannot create context from nil parent") } c := newCancelCtx(parent) propagateCancel(parent, &c) return &c, func() { c.cancel(true, Canceled) } } func newCancelCtx(parent Context) cancelCtx { return cancelCtx{Context: parent} }
上面的方法,我們傳入一個(gè)父 Context(這通常是一個(gè) background,作為根節(jié)點(diǎn)),返回新建的 context,并通過(guò)閉包的形式,返回了一個(gè) cancel 方法。
newCancelCtx將傳入的上下文包裝成私有結(jié)構(gòu)體context.cancelCtx。
propagateCancel則會(huì)構(gòu)建父子上下文之間的關(guān)聯(lián),形成樹(shù)結(jié)構(gòu),當(dāng)父上下文被取消時(shí),子上下文也會(huì)被取消:
func propagateCancel(parent Context, child canceler) { // 1.如果 parent ctx 是不可取消的 ctx,則直接返回 不進(jìn)行關(guān)聯(lián) done := parent.Done() if done == nil { return // parent is never canceled } // 2.接著判斷一下 父ctx 是否已經(jīng)被取消 select { case <-done: // 2.1 如果 父ctx 已經(jīng)被取消了,那就沒(méi)必要關(guān)聯(lián)了 // 然后這里也要順便把子ctx給取消了,因?yàn)楦竎tx取消了 子ctx就應(yīng)該被取消 // 這里是因?yàn)檫€沒(méi)有關(guān)聯(lián)上,所以需要手動(dòng)觸發(fā)取消 // parent is already canceled child.cancel(false, parent.Err()) return default: } // 3. 從父 ctx 中提取出 cancelCtx 并將子ctx加入到父ctx 的 children 里面 if p, ok := parentCancelCtx(parent); ok { p.mu.Lock() // double check 一下,確認(rèn)父 ctx 是否被取消 if p.err != nil { // 取消了就直接把當(dāng)前這個(gè)子ctx給取消了 // parent has already been canceled child.cancel(false, p.err) } else { // 否則就添加到 children 里面 if p.children == nil { p.children = make(map[canceler]struct{}) } p.children[child] = struct{}{} } p.mu.Unlock() } else { // 如果沒(méi)有找到可取消的父 context。新啟動(dòng)一個(gè)協(xié)程監(jiān)控父節(jié)點(diǎn)或子節(jié)點(diǎn)取消信號(hào) atomic.AddInt32(&goroutines, +1) go func() { select { case <-parent.Done(): child.cancel(false, parent.Err()) case <-child.Done(): } }() } }
上面的方法可能遇到以下幾種情況:
當(dāng) parent.Done() == nil,也就是 parent 不會(huì)觸發(fā)取消事件時(shí),當(dāng)前函數(shù)會(huì)直接返回;
當(dāng) child 的繼承鏈包含可以取消的上下文時(shí),會(huì)判斷 parent 是否已經(jīng)觸發(fā)了取消信號(hào);
如果已經(jīng)被取消,child 會(huì)立刻被取消;
如果沒(méi)有被取消,child 會(huì)被加入 parent 的 children 列表中,等待 parent 釋放取消信號(hào);
當(dāng)父上下文是開(kāi)發(fā)者自定義的類(lèi)型、實(shí)現(xiàn)了 context.Context 接口并在 Done()方法中返回了非空的管道時(shí);
運(yùn)行一個(gè)新的 Goroutine 同時(shí)監(jiān)聽(tīng) parent.Done()和 child.Done()兩個(gè) Channel;
在 parent.Done()關(guān)閉時(shí)調(diào)用 child.cancel 取消子上下文;
propagateCancel 的作用是在 parent 和 child 之間同步取消和結(jié)束的信號(hào),保證在 parent 被取消時(shí),child 也會(huì)收到對(duì)應(yīng)的信號(hào),不會(huì)出現(xiàn)狀態(tài)不一致的情況。
func parentCancelCtx(parent Context) (*cancelCtx, bool) { done := parent.Done() // 如果 done 為 nil 說(shuō)明這個(gè)ctx是不可取消的 // 如果 done == closedchan 說(shuō)明這個(gè)ctx不是標(biāo)準(zhǔn)的 cancelCtx,可能是自定義的 if done == closedchan || done == nil { return nil, false } // 然后調(diào)用 value 方法從ctx中提取出 cancelCtx p, ok := parent.Value(&cancelCtxKey).(*cancelCtx) if !ok { return nil, false } // 最后再判斷一下cancelCtx 里存的 done 和 父ctx里的done是否一致 // 如果不一致說(shuō)明parent不是一個(gè) cancelCtx pdone, _ := p.done.Load().(chan struct{}) if pdone != done { return nil, false } return p, true } ancelCtx 的 done 方法會(huì)返回一個(gè) chan struct{}: func (c *cancelCtx) Done() <-chan struct{} { d := c.done.Load() if d != nil { return d.(chan struct{}) } c.mu.Lock() defer c.mu.Unlock() d = c.done.Load() if d == nil { d = make(chan struct{}) c.done.Store(d) } return d.(chan struct{}) } var closedchan = make(chan struct{})
parentCancelCtx 其實(shí)就是判斷 parent context 里面有沒(méi)有一個(gè) cancelCtx,有就返回,讓子context可以“掛靠”到parent context 上,如果不是就返回false,不進(jìn)行掛靠,自己新開(kāi)一個(gè) goroutine 來(lái)監(jiān)聽(tīng)。
3.4 timerCtx
timerCtx 內(nèi)部不僅通過(guò)嵌入 cancelCtx 的方式承了相關(guān)的變量和方法,還通過(guò)持有的定時(shí)器 timer 和截止時(shí)間 deadline 實(shí)現(xiàn)了定時(shí)取消的功能:
type timerCtx struct { cancelCtx timer *time.Timer // Under cancelCtx.mu. deadline time.Time } func (c *timerCtx) Deadline() (deadline time.Time, ok bool) { return c.deadline, true } func (c *timerCtx) cancel(removeFromParent bool, err error) { c.cancelCtx.cancel(false, err) if removeFromParent { removeChild(c.cancelCtx.Context, c) } c.mu.Lock() if c.timer != nil { c.timer.Stop() c.timer = nil } c.mu.Unlock() }
3.5 valueCtx
valueCtx 是多了 key、val 兩個(gè)字段來(lái)存數(shù)據(jù):
type valueCtx struct { Context key, val interface{} }
取值查找的過(guò)程,實(shí)際上是一個(gè)遞歸查找的過(guò)程:
func (c *valueCtx) Value(key interface{}) interface{} { if c.key == key { return c.val } return c.Context.Value(key) }
如果 key 和當(dāng)前 ctx 中存的 value 一致就直接返回,沒(méi)有就去 parent 中找。最終找到根節(jié)點(diǎn)(一般是 emptyCtx),直接返回一個(gè) nil。所以用 Value 方法的時(shí)候要判斷結(jié)果是否為 nil,類(lèi)似于一個(gè)鏈表,效率是很低的,不建議用來(lái)傳參數(shù)。
4. 使用建議
在官方博客里,對(duì)于使用 context 提出了幾點(diǎn)建議:
不要將 Context 塞到結(jié)構(gòu)體里。直接將 Context 類(lèi)型作為函數(shù)的第一參數(shù),而且一般都命名為 ctx
不要向函數(shù)傳入一個(gè) nil 的 context,如果你實(shí)在不知道傳什么,標(biāo)準(zhǔn)庫(kù)給你準(zhǔn)備好了一個(gè) context:todo。
不要把本應(yīng)該作為函數(shù)參數(shù)的類(lèi)型塞到 context 中,context 存儲(chǔ)的應(yīng)該是一些共同的數(shù)據(jù)。例如:登陸的 session、cookie 等。
同一個(gè) context 可能會(huì)被傳遞到多個(gè) goroutine,別擔(dān)心,context 是并發(fā)安全的。
-
接口
+關(guān)注
關(guān)注
33文章
8667瀏覽量
151523 -
數(shù)據(jù)
+關(guān)注
關(guān)注
8文章
7102瀏覽量
89277 -
通信
+關(guān)注
關(guān)注
18文章
6046瀏覽量
136215
原文標(biāo)題:Go 語(yǔ)言上下文 context 底層原理
文章出處:【微信號(hào):magedu-Linux,微信公眾號(hào):馬哥Linux運(yùn)維】歡迎添加關(guān)注!文章轉(zhuǎn)載請(qǐng)注明出處。
發(fā)布評(píng)論請(qǐng)先 登錄
相關(guān)推薦
鴻蒙開(kāi)發(fā)接口Ability框架:【 (Context模塊)】
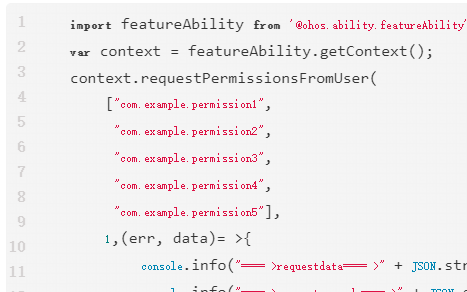
鴻蒙開(kāi)發(fā)接口Ability框架:【Context】
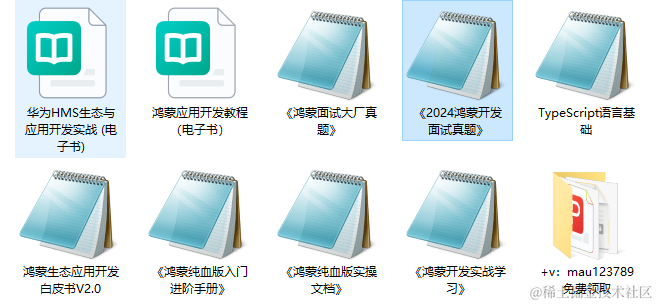
進(jìn)程Context定義
進(jìn)程的Context定義
關(guān)于高阻態(tài)和OOC(out of context)綜合方式
Redis基本類(lèi)型和底層實(shí)現(xiàn)
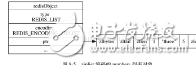
TiDB底層存儲(chǔ)結(jié)構(gòu)LSM樹(shù)原理介紹
In-context learning介紹
介紹下volatile的底層原理
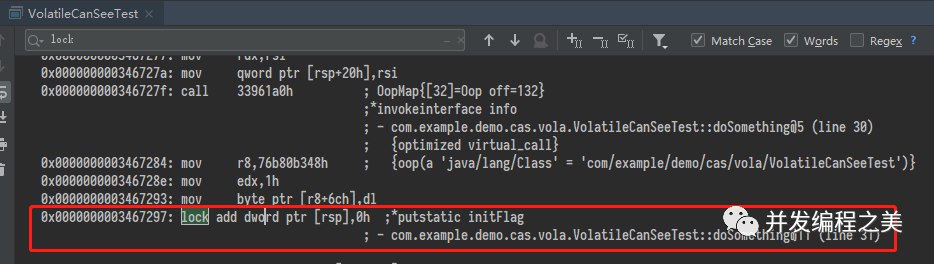
In-Context-Learning在更大的語(yǔ)言模型上表現(xiàn)不同
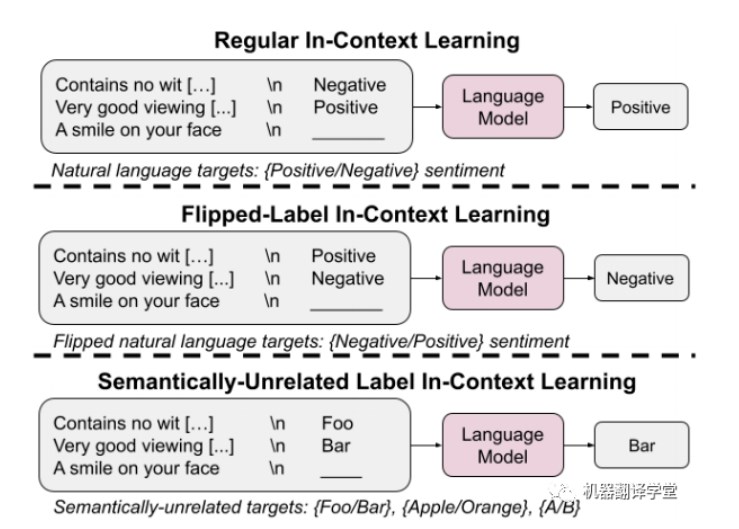
關(guān)于GO CONTEXT機(jī)制實(shí)現(xiàn)原則
鴻蒙開(kāi)發(fā)接口Ability框架:【Context】
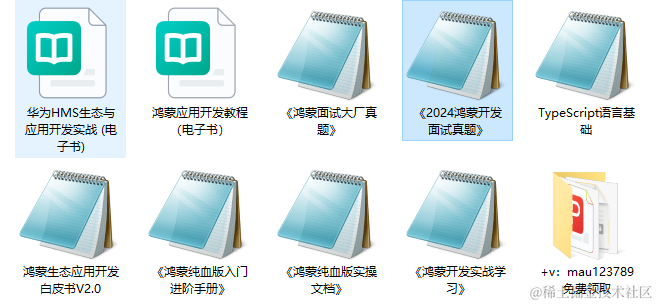
鴻蒙Ability Kit(程序框架服務(wù))【應(yīng)用上下文Context】
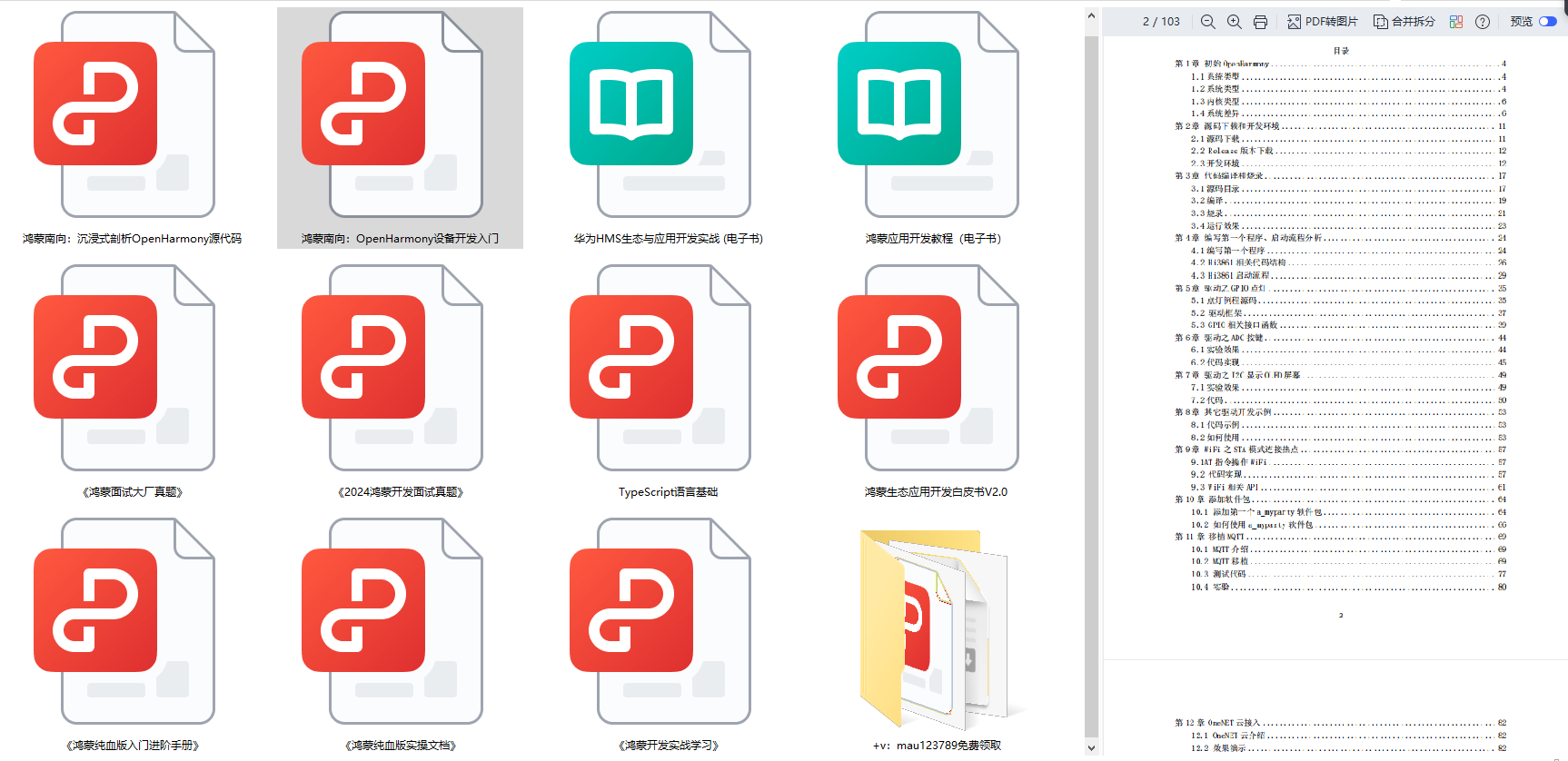
鴻蒙開(kāi)發(fā)組件:FA模型的Context
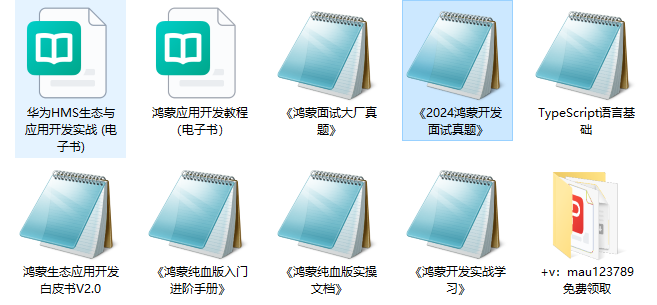
評(píng)論