使用Platformio平臺的libopencm3開發(fā)框架來開發(fā)STM32G0,以下為FreeRTOS和CLI組件使用。
1 新建項目
- 建立freertos_cli項目
在PIO的Home頁面新建項目,項目名稱freertos_cli,選擇開發(fā)板為 MonkeyPi_STM32_G070RB,開發(fā)框架選擇libopencm3;
1upload_protocol = cmsis-dap
2debug_tool = cmsis-dap
2 編寫程序
直接在之前的文章-FreeRTOS基本使用基礎上進行添加,在項目中添加好FreeRTOS源碼后,再將FreeRTOS源碼中的 FreeRTOS-Plus\\Source\\FreeRTOS-Plus-CLI 目錄放置到項目lib目錄下,目錄如下:
image-20220927232611980
2.1 串口設置
1void uart_setup(void)
2{
3
4 //uart pin
5 rcc_periph_clock_enable(RCC_USART1);
6 rcc_periph_clock_enable(RCC_GPIOB);
7
8 gpio_mode_setup(GPIOB,GPIO_MODE_AF,GPIO_PUPD_NONE,GPIO6|GPIO7);
9 gpio_set_af(GPIOB,GPIO_AF0,GPIO6|GPIO7);
10
11 usart_set_baudrate(USART1,115200);
12 usart_set_databits(USART1,8);
13 usart_set_stopbits(USART1,USART_STOPBITS_1);
14 usart_set_parity(USART1,USART_PARITY_NONE);
15 usart_set_flow_control(USART1,USART_FLOWCONTROL_NONE);
16 usart_set_mode(USART1,USART_MODE_TX_RX);
17
18 //uart isr
19 nvic_enable_irq(NVIC_USART1_IRQ);
20
21 usart_enable(USART1);
22
23 usart_enable_rx_interrupt(USART1);
24}
25
26/**
27 * @brief uart1 isr function
28 *
29 */
30void usart1_isr(void)
31{
32 //receive interrupt
33 if (((USART_CR1(USART1) & USART_CR1_RXNEIE) != 0) &&
34 ((USART_ISR(USART1) & USART_ISR_RXNE) != 0)) {
35
36 char c = usart_recv(USART1);
37
38 xQueueSendFromISR(uart_queue, &c, NULL);
39 }
40}
開啟出口中斷,并在串口接收到數(shù)據(jù)時候發(fā)送到隊列;
2.2 命令程序
編寫執(zhí)行命令后要進行的動作函數(shù),這里為了簡單直接打印一個信息:
1static BaseType_t prvHelloCommand(
2 int8_t *pcWriteBuffer,
3 size_t xWriteBufferLen,
4 const int8_t *pcCommandString )
5{
6
7 sprintf(pcWriteBuffer, "hello world command");
8
9 /* Execution of this command is complete, so return pdFALSE. */
10 return pdFALSE;
11}
- pcWriteBuffer 參數(shù)為要寫入的信息;
- xWriteBufferLen 參數(shù)為寫入的緩沖大?。?/li>
- pcCommandString 為整個命令字符串指針,可以使用 FreeRTOS_CLIGetParameter 來獲取命令的參數(shù);
2.3 命令和函數(shù)進行映射
1static const CLI_Command_Definition_t xHelloCommand =
2{
3 "hello",
4 "\\r\\nhello:\\r\\n This is a hello command for testing\\r\\n",
5 prvHelloCommand,
6 0
7};
- 第一個參數(shù)為命令名字;
- 第二個參數(shù)為命令描述;
- 第三個參數(shù)為命令所需要的參數(shù)個數(shù);
2.4 注冊命令
1 //register cli command
2 FreeRTOS_CLIRegisterCommand(&xHelloCommand);
2.5 命令任務
建立一個任務,用于處理CLI輸入輸出:
1static void hello_cli_task(void *args)
2{
3 //register cli command
4 FreeRTOS_CLIRegisterCommand(&xHelloCommand);
5
6 char *outbuff;
7 static int8_t inbuff[64];
8 static int8_t lastinbuff[64];
9 uint8_t index = 0;
10 BaseType_t ret;
11
12 outbuff = FreeRTOS_CLIGetOutputBuffer();
13
14 printf(">");//command prompt
15 fflush(stdout);
16 // printf("\\r\\n");
17
18 char c;
19
20 while(1){
21
22 if( xQueueReceive(uart_queue, &c, 5) == pdPASS) {
23 printf("%c",c);//echo
24 fflush(stdout);
25
26 /* Was it the end of the line? */
27 if( c == '\\n' || c == '\\r' )
28 {
29 printf("\\r\\n");
30 fflush(stdout);
31
32 /* See if the command is empty, indicating that the last command is to be executed again. */
33 if( index == 0 )
34 {
35 /* Copy the last command back into the input string. */
36 strcpy( inbuff, lastinbuff );
37 }
38
39 /* Pass the received command to the command interpreter. The
40 command interpreter is called repeatedly until it returns
41 pdFALSE (indicating there is no more output) as it might
42 generate more than one string. */
43 do
44 {
45 /* Get the next output string from the command interpreter. */
46 ret = FreeRTOS_CLIProcessCommand( inbuff, outbuff, configCOMMAND_INT_MAX_OUTPUT_SIZE );
47
48 /* Write the generated string to the UART. */
49 printf("%s",outbuff);
50 fflush(stdout);
51
52 } while( ret != pdFALSE );
53
54 /* All the strings generated by the input command have been
55 sent. Clear the input string ready to receive the next command.
56 Remember the command that was just processed first in case it is
57 to be processed again. */
58 strcpy( lastinbuff, inbuff );
59 index = 0;
60 memset( inbuff, 0x00, 64 );
61
62 printf("\\r\\n>");
63 fflush(stdout);
64
65 }
66 else
67 {
68 if( c == '\\r' )
69 {
70 /* Ignore the character. */
71 }
72 else if( ( c == '\\b' ) || ( c == 0x7f ) )//del
73 {
74 /* Backspace was pressed. Erase the last character in the
75 string - if any. */
76 if( index > 0 )
77 {
78 index--;
79 inbuff[ index ] = '\\0';
80 }
81 }
82 else
83 {
84 /* A character was entered. Add it to the string entered so
85 far. When a \\n is entered the complete string will be
86 passed to the command interpreter. */
87 if( ( c >= ' ' ) && ( c <= '~' ) )
88 {
89 if( index < 64 )
90 {
91 inbuff[ index ] = c;
92 index++;
93 }
94 }
95 }
96 }
97
98 }
99 }
100
101}
輸入這里直接從之前串口中斷獲取的數(shù)據(jù)隊列中得到;
輸出使用串口打印輸出即可;
3 燒寫測試
將程序燒寫到開發(fā)板,連接好串口后,執(zhí)行命令測試:
image-20220928203000256
-
STM32
+關注
關注
2270文章
10900瀏覽量
355927 -
串口
+關注
關注
14文章
1554瀏覽量
76497 -
開發(fā)板
+關注
關注
25文章
5047瀏覽量
97442 -
FreeRTOS
+關注
關注
12文章
484瀏覽量
62166 -
CLI
+關注
關注
1文章
79瀏覽量
8543
發(fā)布評論請先 登錄
相關推薦
STM32G0開發(fā)筆記:FreeRTOS和FreeModbus庫使用
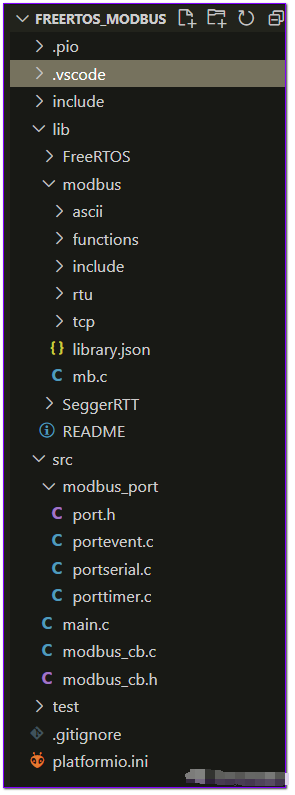
STM32G0開發(fā)筆記:使用FreeRTOS系統(tǒng)的隊列Queue
STM32G0開發(fā)筆記:使用FreeRTOS系統(tǒng)
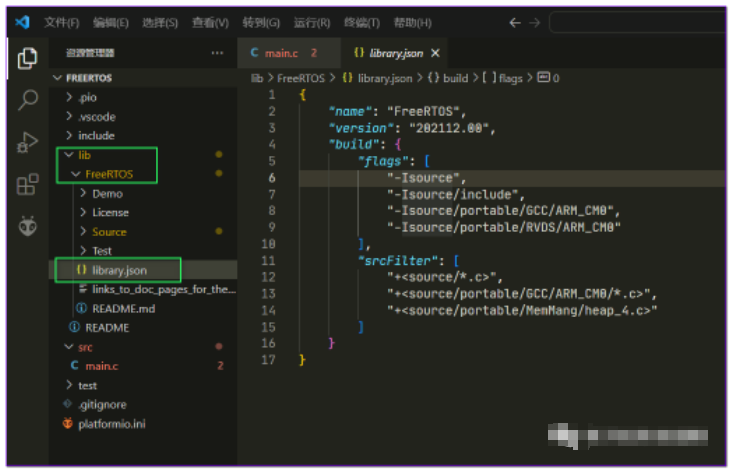
STM32G0開發(fā)筆記:用PWM來實現(xiàn)LED呼吸燈效果

STM32G0開發(fā)筆記:EEPROM M24C02的使用方法
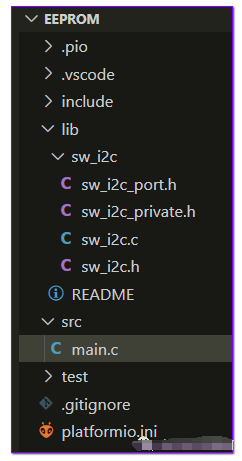
STM32G0開發(fā)筆記:串口中斷的使用
STM32G0開發(fā)筆記:GPIO接按鍵的使用方式
STM32G0開發(fā)筆記:使用libopencm3庫
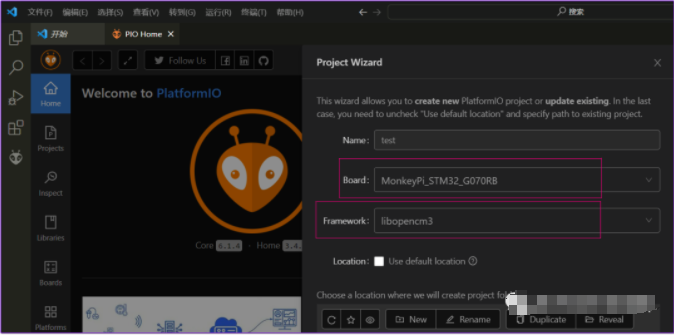
評論