一、簡介
基于 SpringBoot 平臺開發的項目數不勝數,與常規的基于Spring
開發的項目最大的不同之處,SpringBoot 里面提供了大量的注解用于快速開發,而且非常簡單,基本可以做到開箱即用!
那 SpringBoot 為開發者提供了多少注解呢?我們該如何使用?
針對此問題,小編特意對其進行了一番整理,內容如下,個人感覺還是比較清晰的,今天我們就一起來整一整每個注解的含義和用法,以免踩坑!
二、注解總結
2.1、SpringMVC 相關注解
@Controller
通常用于修飾controller
層的組件,由控制器負責將用戶發來的URL
請求轉發到對應的服務接口,通常還需要配合注解@RequestMapping
使用。
@RequestMapping
提供路由信息,負責URL
到Controller
中具體函數的映射,當用于方法上時,可以指定請求協議,比如GET
、POST
、PUT
、DELETE
等等。
@RequestBody
表示請求體的Content-Type
必須為application/json
格式的數據,接收到數據之后會自動將數據綁定到Java
對象上去
@ResponseBody
表示該方法的返回結果直接寫入HTTP response body
中,返回數據的格式為application/json
比如,請求參數為json
格式,返回參數也為json
格式,示例代碼如下:
/**
* 登錄服務
*/
@Controller
@RequestMapping("api")
public class LoginController {
/**
* 登錄請求,post請求協議,請求參數數據格式為json
* @param request
*/
@RequestMapping(value = "login", method = RequestMethod.POST)
@ResponseBody
public ResponseEntity login(@RequestBody UserLoginDTO request){
//...業務處理
return new ResponseEntity(HttpStatus.OK);
}
}
@RestController
和@Controller
一樣,用于標注控制層組件,不同的地方在于:它是@ResponseBody
和@Controller
的合集,也就是說,在當@RestController
用在類上時,表示當前類里面所有對外暴露的接口方法,返回數據的格式都為application/json
,示范代碼如下:
@RestController
@RequestMapping("api")
public class LoginController {
/**
* 登錄請求,post請求協議,請求參數數據格式為json
* @param request
*/
@RequestMapping(value = "login", method = RequestMethod.POST)
public ResponseEntity login(@RequestBody UserLoginDTO request){
//...業務處理
return new ResponseEntity(HttpStatus.OK);
}
}
@RequestParam
用于接收請求參數為表單類型的數據,通常用在方法的參數前面,示范代碼如下:
/**
* 登錄請求,post請求協議,請求參數數據格式為表單
*/
@RequestMapping(value = "login", method = RequestMethod.POST)
@ResponseBody
public ResponseEntity login(@RequestParam(value = "userName",required = true) String userName,
@RequestParam(value = "userPwd",required = true) String userPwd){
//...業務處理
return new ResponseEntity(HttpStatus.OK);
}
@PathVariable
用于獲取請求路徑中的參數,通常用于restful
風格的api
上,示范代碼如下:
/**
* restful風格的參數請求
* @param id
*/
@RequestMapping(value = "queryProduct/{id}", method = RequestMethod.POST)
@ResponseBody
public ResponseEntity queryProduct(@PathVariable("id") String id){
//...業務處理
return new ResponseEntity(HttpStatus.OK);
}
@GetMapping
除了@RequestMapping
可以指定請求方式之外,還有一些其他的注解,可以用于標注接口路徑請求,比如GetMapping
用在方法上時,表示只支持get
請求方法,等價于@RequestMapping(value="/get",method=RequestMethod.GET)
@GetMapping("get")
public ResponseEntity get(){
return new ResponseEntity(HttpStatus.OK);
}
@PostMapping
用在方法上,表示只支持post
方式的請求。
@PostMapping("post")
public ResponseEntity post(){
return new ResponseEntity(HttpStatus.OK);
}
@PutMapping
用在方法上,表示只支持put
方式的請求,通常表示更新某些資源的意思
@PutMapping("put")
public ResponseEntity put(){
return new ResponseEntity(HttpStatus.OK);
}
@DeleteMapping
用在方法上,表示只支持delete
方式的請求,通常表示刪除某些資源的意思
@DeleteMapping("delete")
public ResponseEntity delete(){
return new ResponseEntity(HttpStatus.OK);
}
2.2、bean 相關注解
@Service
通常用于修飾service
層的組件,聲明一個對象,會將類對象實例化并注入到bean
容器里面
@Service
public class DeptService {
//具體的方法
}
@Component
泛指組件,當組件不好歸類的時候,可以使用這個注解進行標注,功能類似于于@Service
@Component
public class DeptService {
//具體的方法
}
@Repository
通常用于修飾dao
層的組件,
@Repository
注解屬于Spring
里面最先引入的一批注解,它用于將數據訪問層 (DAO
層 ) 的類標識為Spring Bean
,具體只需將該注解標注在 DAO類上即可,示例代碼如下:
@Repository
public interface RoleRepository extends JpaRepository<Role,Long> {
//具體的方法
}
為什么現在使用的很少呢?
主要是因為當我們配置服務啟動自動掃描dao
層包時,Spring
會自動幫我們創建一個實現類,然后注入到bean
容器里面。當某些類無法被掃描到時,我們可以顯式的在數據持久類上標注@Repository
注解,Spring
會自動幫我們聲明對象。
@Bean
相當于 xml 中配置 Bean,意思是產生一個 bean 對象,并交給spring管理,示例代碼如下:
@Configuration
public class AppConfig {
//相當于 xml 中配置 Bean
@Bean
public Uploader initFileUploader() {
return new FileUploader();
}
}
@Autowired
自動導入依賴的bean
對象,默認時按照byType
方式導入對象,而且導入的對象必須存在,當需要導入的對象并不存在時,我們可以通過配置required = false
來關閉強制驗證。
@Autowired
private DeptService deptService;
@Resource
也是自動導入依賴的bean
對象, 由JDK
提供 ,默認是按照byName
方式導入依賴的對象;而@Autowired
默認時按照byType
方式導入對象,當然@Resource
還可以配置成通過byType
方式導入對象。
/**
* 通過名稱導入(默認通過名稱導入依賴對象)
*/
@Resource(name = "deptService")
private DeptService deptService;
/**
* 通過類型導入
*/
@Resource(type = RoleRepository.class)
private DeptService deptService;
@Qualifier
當有多個同一類型的bean
時,使用@Autowired
導入會報錯,提示當前對象并不是唯一,Spring
不知道導入哪個依賴,這個時候,我們可以使用@Qualifier
進行更細粒度的控制,選擇其中一個候選者,一般于@Autowired
搭配使用,示例如下:
@Autowired
@Qualifier("deptService")
private DeptService deptService;
@Scope
用于生命一個spring bean
的作用域,作用的范圍一共有以下幾種:
- singleton:唯一 bean 實例,Spring 中的 bean 默認都是單例的。
- prototype:每次請求都會創建一個新的 bean 實例,對象多例。
- request:每一次 HTTP 請求都會產生一個新的 bean,該 bean 僅在當前 HTTP request 內有效。
- session:每一次 HTTP 請求都會產生一個新的 bean,該 bean 僅在當前 HTTP session 內有效。
/**
* 單例對象
*/
@RestController
@Scope("singleton")
public class HelloController {
}
-
spring
+關注
關注
0文章
340瀏覽量
14343 -
MVC
+關注
關注
0文章
73瀏覽量
13857 -
開發者
+關注
關注
1文章
575瀏覽量
17009 -
SpringBoot
+關注
關注
0文章
173瀏覽量
178
發布評論請先 登錄
相關推薦
SpringBoot應用啟動運行run方法
HarmonyOS注解的使用方法分享
Spring Boot常用注解與使用方式
Java注解及其底層原理解析 1
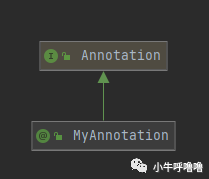
SpringBoot常用注解及使用方法2
Springboot常用注解合集

評論