數(shù)字低通濾波器模塊,做平滑濾波
//*********************************************************************
//
// 'Box' Average
//
// Standard Mean Average Calculation
// Can be modeled as FIR Low-Pass Filter where
// all coefficients are equal to '1'.
//
//*********************************************************************
module box_ave (
clk,
rstn,
sample,
raw_data_in,
ave_data_out,
data_out_valid);
parameter
ADC_WIDTH = 8, // ADC Convertor Bit Precision
LPF_DEPTH_BITS = 4; // 2^LPF_DEPTH_BITS is decimation rate of averager
//input ports
input clk; // sample rate clock
input rstn; // async reset, asserted low
input sample; // raw_data_in is good on rising edge,
input [ADC_WIDTH-1:0] raw_data_in; // raw_data input
//output ports
output [ADC_WIDTH-1:0] ave_data_out; // ave data output
output data_out_valid; // ave_data_out is valid, single pulse
reg [ADC_WIDTH-1:0] ave_data_out;
//**********************************************************************
//
// Internal Wire & Reg Signals
//
//**********************************************************************
reg [ADC_WIDTH+LPF_DEPTH_BITS-1:0] accum; // accumulator
reg [LPF_DEPTH_BITS-1:0] count; // decimation count
reg [ADC_WIDTH-1:0] raw_data_d1; // pipeline register
reg sample_d1, sample_d2; // pipeline registers
reg result_valid; // accumulator result 'valid'
wire accumulate; // sample rising edge detected
wire latch_result; // latch accumulator result
//***********************************************************************
//
// Rising Edge Detection and data alignment pipelines
//
//***********************************************************************
always @(posedge clk or negedge rstn)
begin
if( ~rstn ) begin
sample_d1 <= 0;
sample_d2 <= 0;
raw_data_d1 <= 0;
result_valid <= 0;
end else begin
sample_d1 <= sample; // capture 'sample' input
sample_d2 <= sample_d1; // delay for edge detection
raw_data_d1 <= raw_data_in; // pipeline
result_valid <= latch_result; // pipeline for alignment with result
end
end
assign accumulate = sample_d1 && !sample_d2; // 'sample' rising_edge detect
assign latch_result = accumulate && (count == 0); // latch accum. per decimation count
//***********************************************************************
//
// Accumulator Depth counter
//
//***********************************************************************
always @(posedge clk or negedge rstn)
begin
if( ~rstn ) begin
count <= 0;
end else begin
if (accumulate) count <= count + 1; // incr. count per each sample
end
end
//***********************************************************************
//
// Accumulator
//
//***********************************************************************
always @(posedge clk or negedge rstn)
begin
if( ~rstn ) begin
accum <= 0;
end else begin
if (accumulate)
if(count == 0) // reset accumulator
accum <= raw_data_d1; // prime with first value
else
accum <= accum + raw_data_d1; // accumulate
end
end
//***********************************************************************
//
// Latch Result
//
// ave = (summation of 'n' samples)/'n' is right shift when 'n' is power of two
//
//***********************************************************************
always @(posedge clk or negedge rstn)
begin
if( ~rstn ) begin
ave_data_out <= 0;
end else if (latch_result) begin // at end of decimation period...
ave_data_out <= accum >> LPF_DEPTH_BITS; // ... save accumulator/n result
end
end
assign data_out_valid = result_valid; // output assignment
endmodule
有FPGA的小朋友不妨可以試一下,只需要搭配一顆比較器、一個(gè)電阻R、一個(gè)電容C,只需要FPGA的兩根管腳,你就可以擁有ADC的功能了。
聲明:本文內(nèi)容及配圖由入駐作者撰寫或者入駐合作網(wǎng)站授權(quán)轉(zhuǎn)載。文章觀點(diǎn)僅代表作者本人,不代表電子發(fā)燒友網(wǎng)立場(chǎng)。文章及其配圖僅供工程師學(xué)習(xí)之用,如有內(nèi)容侵權(quán)或者其他違規(guī)問(wèn)題,請(qǐng)聯(lián)系本站處理。
舉報(bào)投訴
-
FPGA
+關(guān)注
關(guān)注
1629文章
21748瀏覽量
603954 -
芯片
+關(guān)注
關(guān)注
456文章
50889瀏覽量
424302 -
adc
+關(guān)注
關(guān)注
98文章
6505瀏覽量
544904
發(fā)布評(píng)論請(qǐng)先 登錄
相關(guān)推薦
fpga開(kāi)發(fā)板使用教程之在K7上用Ibert實(shí)現(xiàn)基本的GTX測(cè)試
測(cè)試,而且基本上可以達(dá)到不用敲代碼就可以完成測(cè)試的目的。 下面按步驟,一步一步實(shí)現(xiàn)。重點(diǎn)的地方我
發(fā)表于 12-31 15:36
?7886次閱讀
ADC實(shí)現(xiàn)一個(gè)IO上掛多個(gè)按鍵
有時(shí)候做設(shè)計(jì)時(shí),我們會(huì)遇到外部按鍵比較多,IO口不夠用的情況。這時(shí)大部分人會(huì)考慮通過(guò)其它芯片擴(kuò)展IO,或者直接換一個(gè)IO口足夠的MCU。其實(shí),還有個(gè)方法可以
發(fā)表于 09-01 13:25
?2996次閱讀
如何用STM32F407實(shí)現(xiàn)一個(gè)可編程的模擬比較器的功能?
STM32F407沒(méi)有獨(dú)立的模擬比較端口,如何能實(shí)現(xiàn)一個(gè)可編程的模擬比較器
發(fā)表于 11-28 14:00
STM32F303VCT6比較器只有三個(gè)可以用
STM32F303VCT6共有7個(gè)比較器,為何只有COMP1、COMP2、COMP7這三個(gè)可以用
發(fā)表于 02-13 07:13
基于Ginkgo 2的ADC實(shí)現(xiàn)虛擬示波器功能
模塊,調(diào)用ADC_Init()函數(shù)即可,詳細(xì)調(diào)用方法請(qǐng)參考程序源碼。2、開(kāi)一個(gè)定時(shí)器實(shí)現(xiàn)定時(shí)讀取
發(fā)表于 02-21 11:38
怎么利用FPGA和CPLD數(shù)字邏輯實(shí)現(xiàn)ADC?
數(shù)字系統(tǒng)的設(shè)計(jì)人員擅長(zhǎng)在其印制電路板上用FPGA和CPLD將各種處理器、存儲(chǔ)器和標(biāo)準(zhǔn)的功能元件粘
發(fā)表于 08-19 06:15
如何利用FPGA實(shí)現(xiàn)高頻率ADC?
數(shù)字系統(tǒng)的設(shè)計(jì)人員擅長(zhǎng)在其印制電路板上用FPGA和CPLD將各種處理器、存儲(chǔ)器和標(biāo)準(zhǔn)的功能元件粘
發(fā)表于 09-19 06:18
可以用FPGA做些什么
我剛買了新的NEXYS4 DDR板,我已經(jīng)安裝并使用了Vivado套件。我的問(wèn)題是我可以用FPGA做些什么?我是一名計(jì)算機(jī)/電氣學(xué)生,我之所以采用這種邏輯與實(shí)驗(yàn)課是因?yàn)槲乙呀?jīng)參加了我學(xué)校提供的常規(guī)
發(fā)表于 05-05 07:28
我可以用STM32實(shí)現(xiàn)什么?為什么使用STM32而不是8051
你問(wèn),如何系統(tǒng)地入門學(xué)習(xí)STM32?本身就是一個(gè)錯(cuò)誤的問(wèn)題。假如你會(huì)使用8051 , 會(huì)寫C語(yǔ)言,那么STM32本身并不需要刻意的學(xué)習(xí)。你要考慮的是, 我可以用STM32實(shí)現(xiàn)
發(fā)表于 02-25 06:43
用matlab來(lái)實(shí)現(xiàn)fpga功能的設(shè)計(jì)
用matlab來(lái)實(shí)現(xiàn)fpga功能的設(shè)計(jì)
摘要:System Generator for DSP是Xilinx公司開(kāi)發(fā)的基于Matlab的DSP開(kāi)發(fā)工具?熗?時(shí)也是
發(fā)表于 01-16 18:10
?1.1w次閱讀
基于FPGA和CPLD數(shù)字邏輯實(shí)現(xiàn)ADC技術(shù)
基于FPGA和CPLD數(shù)字邏輯實(shí)現(xiàn)ADC技術(shù)
數(shù)字系統(tǒng)的設(shè)計(jì)人員擅長(zhǎng)在其印制電路板上用FPGA
發(fā)表于 05-25 09:39
?1454次閱讀
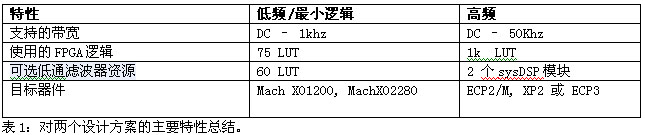
基于fpga和cpld低頻/最小邏輯ADC實(shí)現(xiàn)
數(shù)字系統(tǒng)的設(shè)計(jì)人員擅長(zhǎng)在其印制電路板上用FPGA和CPLD將各種處理器、存儲(chǔ)器和標(biāo)準(zhǔn)的功能元件粘
發(fā)表于 04-26 11:53
?1389次閱讀
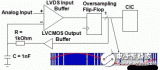
編寫一個(gè)可以用GRUB來(lái)引導(dǎo)的簡(jiǎn)單x86內(nèi)核
我們將從零開(kāi)始,動(dòng)手編寫一個(gè)可以用GRUB來(lái)引導(dǎo)的簡(jiǎn)單x86內(nèi)核,該內(nèi)核會(huì)在屏幕上打印一條信息,然后——掛起!
如何制作一個(gè)簡(jiǎn)易的Sigma Delta ADC?
本文為備戰(zhàn)電賽的案例之一,涉及到的知識(shí)技能: FPGA的使用 ADC的原理及構(gòu)成 PWM的產(chǎn)生 比較器的應(yīng)用 數(shù)字濾波
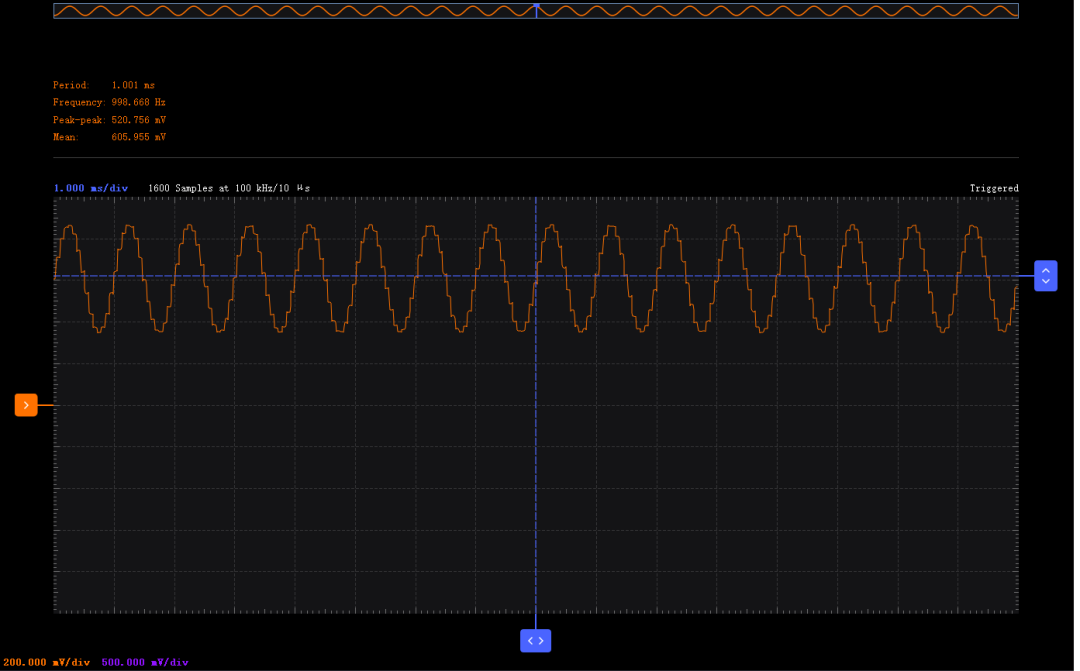
評(píng)論