NVIDIA TensorRT-LLM 是一個專為優(yōu)化大語言模型 (LLM) 推理而設計的庫。它提供了多種先進的優(yōu)化技術,包括自定義 Attention Kernel、Inflight Batching、Paged KV Caching、量化技術 (FP8、INT4 AWQ、INT8 SmoothQuant 等) 以及更多功能,確保您的 NVIDIA GPU 能發(fā)揮出卓越的推理性能。
我們深知您對易用性的需求,為了讓您更快上手,并迅速實現(xiàn)流行模型的高性能推理,我們開發(fā)了 LLM API,通過簡潔的指令,您可輕松體驗 TensorRT-LLM 帶來的卓越性能!
LLM API 是一個 high-level Python API,專為 LLM 推理工作流而設計。以下是一個展示如何使用 TinyLlama 的簡單示例:
from tensorrt_llm import LLM, SamplingParams prompts = [ "Hello, my name is", "The president of the United States is", "The capital of France is", "The future of AI is", ] sampling_params = SamplingParams(temperature=0.8, top_p=0.95) llm = LLM(model="TinyLlama/TinyLlama-1.1B-Chat-v1.0") outputs = llm.generate(prompts, sampling_params) # Print the outputs. for output in outputs: prompt = output.prompt generated_text = output.outputs[0].text print(f"Prompt:{prompt!r},Generatedtext:{generated_text!r}")
希望以上示例能幫助您快速入門 NVIDIA TensorRT-LLM LLM API。
當前 TensorRT-LLM LLM API 可支持的模型
Llama (including variants Mistral, Mixtral, InternLM)
GPT (including variants Starcoder-1/2, Santacoder)
Gemma-1/2
Phi-1/2/3
ChatGLM (including variants glm-10b, chatglm, chatglm2, chatglm3, glm4)
QWen-1/1.5/2
Falcon
Baichuan-1/2
GPT-J
Mamba-1/2
一、詳細介紹
1.1 模型準備
LLM class 可支持以下三種模型導入來源:
Hugging Face Hub:直接從 Hugging Face 模型庫下載模型,例如 TinyLlama/TinyLlama-1.1B-Chat-v1.0。
本地 Hugging Face 模型:使用已下載到本地的 Hugging Face 模型。
本地 TensorRT-LLM 引擎:使用通過 trtllm-build 工具構建或由 Python LLM API 保存的 Engine。
您可以使用 LLM(model=
Hugging Face Hub
使用 Hugging Face Hub 來導入模型非常直觀,只需在 LLM 構造函數(shù)中指定模型倉庫名稱即可:
llm = LLM(model="TinyLlama/TinyLlama-1.1B-Chat-v1.0")
本地 Hugging Face 模型
由于 Hugging Face 模型庫的廣泛應用,API 將 Hugging Face 格式作為主要輸入來源之一。當您要使用 Llama 3.1 模型時,請通過以下命令從 Meta Llama 3.1 8B 模型頁面下載模型:
git lfs install gitclone
下載完成后,您可以通過以下方式加載模型:
llm = LLM(model=)
請注意,使用此模型需要遵守特定許可條款:
https://ai.meta.com/resources/models-and-libraries/llama-downloads/
在開始下載之前,請確保同意這些條款并在 Hugging Face 上完成身份驗證:
https://huggingface.co/meta-llama/Meta-Llama-3-8B?clone=true
本地 TensorRT-LLM 引擎
LLM API 支持使用 TensorRT-LLM Engine,您可以通過以下兩種方式構建 Engine:
您可使用 trtllm-build 工具從 Hugging Face 模型直接構建 TensorRT-LLM Engine,并將其保存到磁盤供后續(xù)使用。詳細說明請參考 GitHub 上的 README 和 examples/llama 倉庫。構建完成后,您可以這樣加載模型:
llm = LLM(model=)
或者,您可以使用 LLM instance 來創(chuàng)建 Engine 并保存到本地磁盤:
llm = LLM() # Save engine to local disk llm.save( )
可以參考第一種方法使用 model 參數(shù)來加載 Engine。
1.2 使用技巧和故障排除
以下是針對熟悉 TensorRT-LLM 其他 API 的用戶,在剛開始使用 LLM API 時可能遇到的常見問題及其解決方案:
RuntimeError: only rank 0 can start multi-node session, got 1
在使用 LLM API 進行單節(jié)點多 GPU 推理時,無需添加 mpirun 前綴。您可以直接運行 python llm_inference_distributed.py 來執(zhí)行多 GPU 推理。
Slurm 節(jié)點上的掛起問題
在使用 Slurm 管理的節(jié)點上遇到掛起或其他問題時,請在啟動腳本中添加前綴 mpirun -n 1 --oversubscribe --allow-run-as-root。
示例命令:
mpirun-n1--oversubscribe--allow-run-as-rootpythonllm_inference_distributed.py
在通訊器 MPI_COMM_WORLD 中,MPI_ABORT 在 rank 1 上被調(diào)用,錯誤代碼為 1。
由于 LLM API 依賴 mpi4py 庫,為避免 mpi4py 中的遞歸生成進程,請將 LLM 類放在函數(shù)中,并在 __main__ 命名空間下保護程序的主入口點。
注意:此限制僅適用于多 GPU 推理。
二、常見自定義操作
2.1 量化
TensorRT-LLM 可以通過在 LLM 實例中設置適當 Flags,自動對 Hugging Face 模型進行量化。例如,要執(zhí)行 Int4 AWQ 量化,以下代碼會觸發(fā)模型量化。請參考完整的支持的標志列表和可接受的值。
fromtensorrt_llm.llmapiimportQuantConfig,QuantAlgo quant_config = QuantConfig(quant_algo=QuantAlgo.W4A16_AWQ) llm=LLM(,quant_config=quant_config)
2.2 采樣
SamplingParams 可以自定義采樣策略以控制 LLM 生成的響應,如 Beam Search、Temperature 和其他參數(shù)。
例如,要啟用 Beam Size 為 4 的 Beam Search,請按如下方式設置 Sampling_Params:
from tensorrt_llm.llmapi import LLM, SamplingParams, BuildConfig build_config = BuildConfig() build_config.max_beam_width = 4 llm = LLM(, build_config=build_config) # Let the LLM object generate text with the default sampling strategy, or # you can create a SamplingParams object as well with several fields set manually sampling_params = SamplingParams(beam_width=4) # current limitation: beam_width should be equal to max_beam_width for output in llm.generate( , sampling_params=sampling_params): print(output)
SamplingParams 管理并分發(fā)字段到 C++ classes,包括:
SamplingConfig:
https://nvidia.github.io/TensorRT-LLM/_cpp_gen/runtime.html#_CPPv4N12tensorrt_llm7runtime14SamplingConfigE
OutputConfig:
https://nvidia.github.io/TensorRT-LLM/_cpp_gen/executor.html#_CPPv4N12tensorrt_llm8executor12OutputConfigE
更多詳情請參考 class 文檔:
https://nvidia.github.io/TensorRT-LLM/llm-api/reference.html#tensorrt_llm.llmapi.SamplingParams
2.3 Build 配置
除了上述參數(shù)外,您還可以使用 build_config 類和從 trtllm-build CLI 借用的其他參數(shù)來自定義構建配置。這些構建配置選項為目標硬件和用例構建 Engine 提供了靈活性。請參考以下示例:
11m = LLM(, build_config=Buildconfig( max_num_tokens=4096, max batch size=128, max_beam_width=4))
?
更多詳情請參考 buildconfig 文檔:
https://github.com/NVIDIA/TensorRT-LLM/blob/main/tensorrt_llm/builder.py#L476-L509
2.4 自定義 Runtime
類似于 build_config,您也可以使用 runtime_config、peft_cache_config,或其他從 Executor API 借用的參數(shù)來自定義 Runtime 配置。這些 Runtime 配置選項在 KV cache management、GPU memory allocation 等方面提供了額外的靈活性。請參考以下示例:
fromtensorrt_llm.llmapiimportLLM,KvCacheConfig llm=LLM(, kv_cache_config=KvCacheConfig( free_gpu_memory_fraction=0.8))
2.5 自定義 Tokenizer
默認情況下,LLM API 使用 transformers 的 AutoTokenizer。您可以在創(chuàng)建 LLM 對象時傳入自己的分詞器來覆蓋它。請參考以下示例:
llm=LLM(,tokenizer= )
?
LLM () 工作流將使用您的 tokenizer 。
也可以使用以下代碼直接輸入 token ID,由于未使用 Tokenizers,該代碼生成的是不帶文本的 token ID。
llm=LLM() foroutputinllm.generate([32,12]): ...
關于作者
嚴春偉
NVIDIA 性能架構師,目前主要聚焦于大模型推理架構和優(yōu)化。
張國銘
NVIDIA 性能架構師,目前主要從事大模型推理架構和優(yōu)化 。
Adam Zheng
NVIDIA 產(chǎn)品經(jīng)理,負責 NVIDIA AI 平臺軟件產(chǎn)品管理,目前主要聚焦于大模型推理架構和優(yōu)化。
-
NVIDIA
+關注
關注
14文章
4986瀏覽量
103047 -
gpu
+關注
關注
28文章
4739瀏覽量
128941 -
模型
+關注
關注
1文章
3243瀏覽量
48836 -
LLM
+關注
關注
0文章
288瀏覽量
334
原文標題:TensorRT-LLM: LLM API 精簡指令暢享卓越性能!
文章出處:【微信號:NVIDIA-Enterprise,微信公眾號:NVIDIA英偉達企業(yè)解決方案】歡迎添加關注!文章轉(zhuǎn)載請注明出處。
發(fā)布評論請先 登錄
相關推薦
如何在Windows電腦電源選項開啟“卓越性能”模式呢
卓越性能電源計劃的目的
查看開啟卓越性能模式前的系統(tǒng)各信息
高性能選項和卓越性能基礎知識總結(jié)
阿里云 & NVIDIA TensorRT Hackathon 2023 決賽圓滿收官,26 支 AI 團隊嶄露頭角
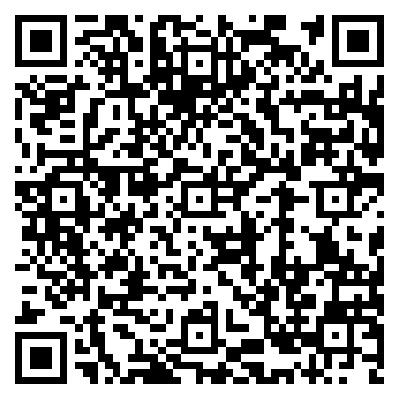
現(xiàn)已公開發(fā)布!歡迎使用 NVIDIA TensorRT-LLM 優(yōu)化大語言模型推理
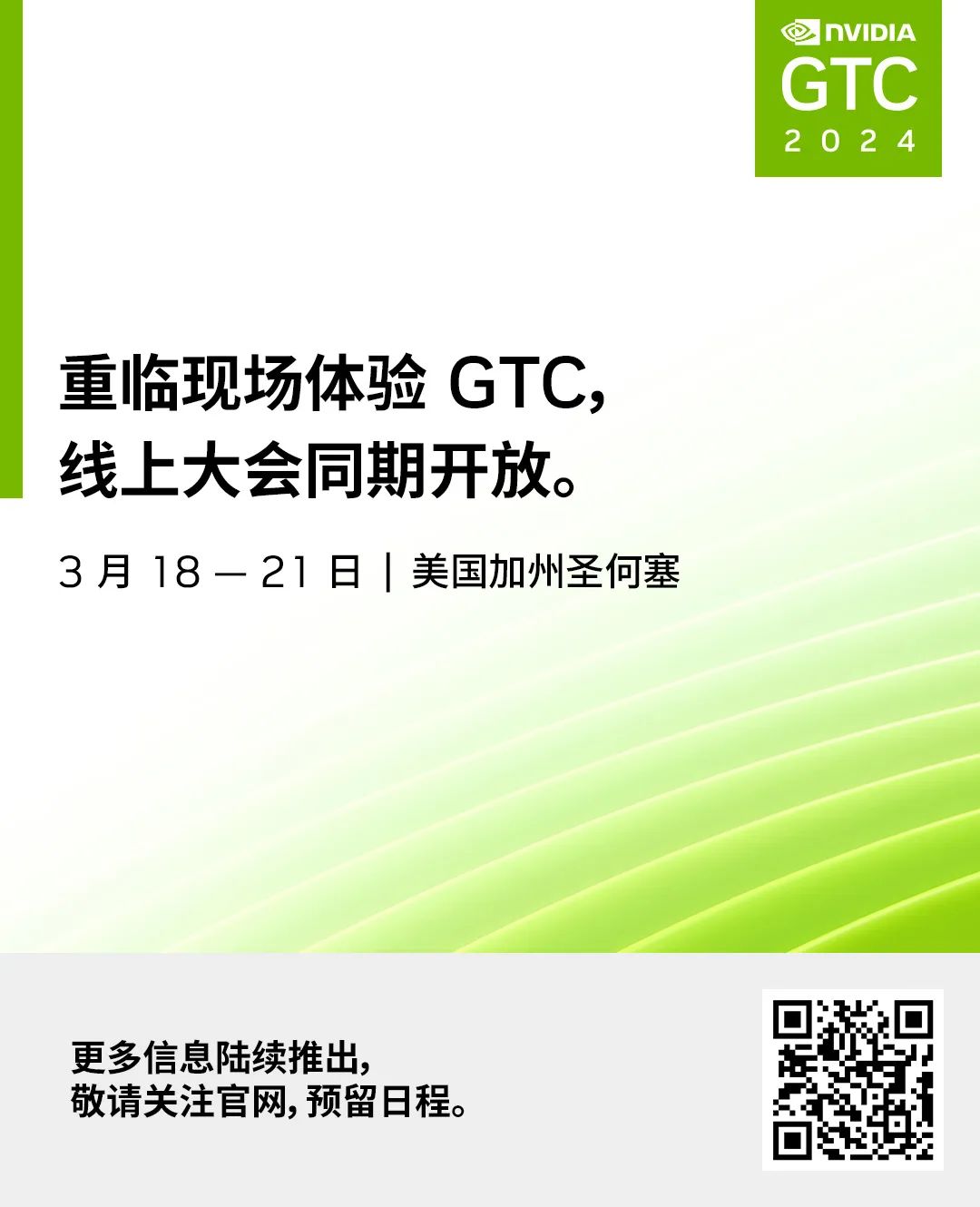
點亮未來:TensorRT-LLM 更新加速 AI 推理性能,支持在 RTX 驅(qū)動的 Windows PC 上運行新模型
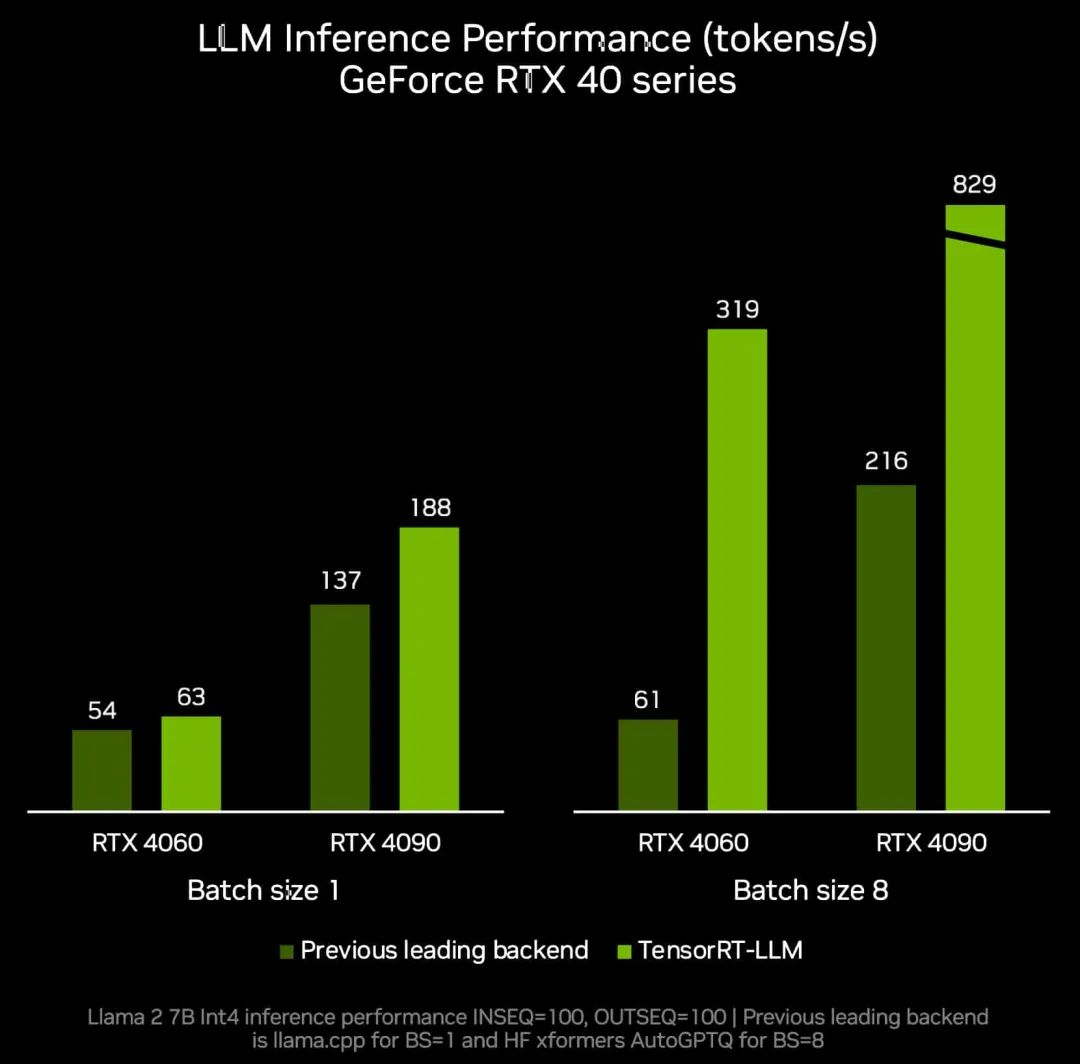
如何在 NVIDIA TensorRT-LLM 中支持 Qwen 模型
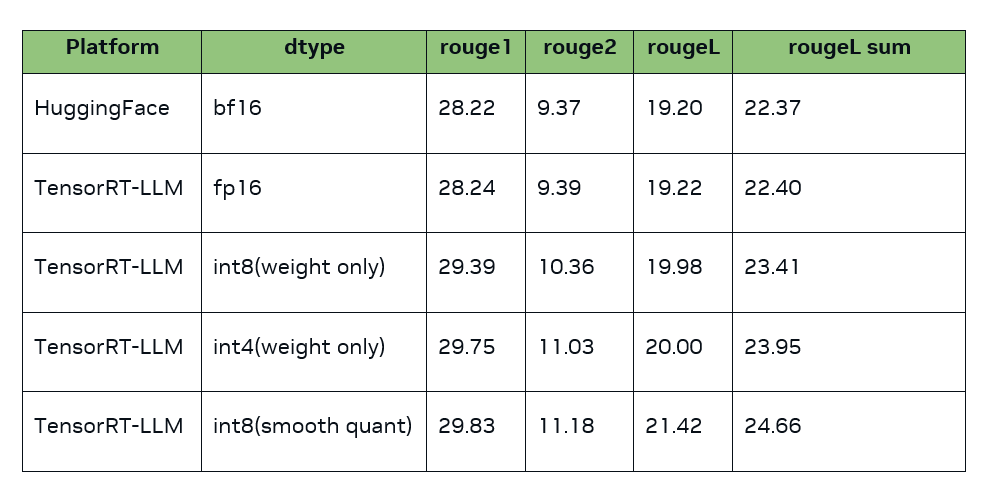
NVIDIA加速微軟最新的Phi-3 Mini開源語言模型
魔搭社區(qū)借助NVIDIA TensorRT-LLM提升LLM推理效率
NVIDIA Nemotron-4 340B模型幫助開發(fā)者生成合成訓練數(shù)據(jù)
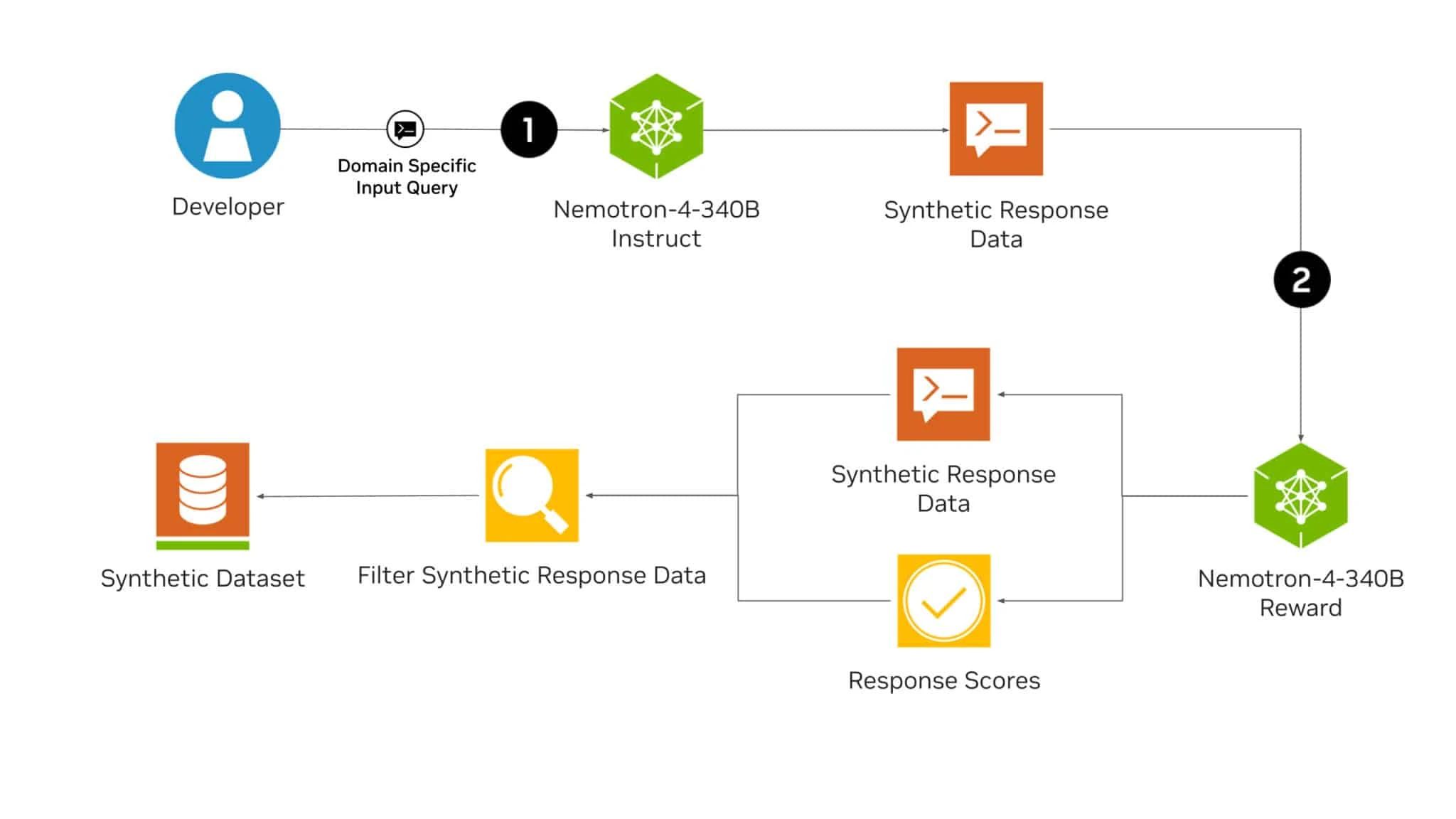
TensorRT-LLM低精度推理優(yōu)化
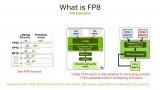
NVIDIA TensorRT-LLM Roadmap現(xiàn)已在GitHub上公開發(fā)布
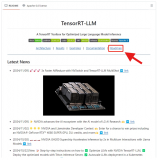
在NVIDIA TensorRT-LLM中啟用ReDrafter的一些變化
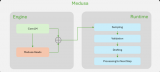
評論